您所在的位置:首页 - 百科 - 正文百科
平板编写代码
承歆
05-17
【百科】
53人已围观
摘要**Title:GettingStartedwithPHPDevelopmentforWebApplications**PHP(HypertextPreprocessor)isapowerfulser
Title: Getting Started with PHP Development for Web Applications
PHP (Hypertext Preprocessor) is a powerful serverside scripting language widely used for web development. Its versatility and ease of integration with HTML make it a popular choice for creating dynamic and interactive websites. If you're interested in diving into PHP development for web applications, here's a comprehensive guide to get you started.
Understanding PHP
PHP is a serverside scripting language, meaning the code is executed on the server before being sent to the client's browser. This allows PHP to generate dynamic content, interact with databases, handle forms, and perform various other tasks necessary for web development.
Setting Up Your Development Environment
1.
Install a Web Server
: You'll need a web server to interpret PHP code. Popular choices include Apache, Nginx, and LiteSpeed. XAMPP and WampServer are bundled solutions that include Apache, MySQL, PHP, and other tools in a single package, making setup easy for beginners.2.
Install PHP
: Download and install the latest version of PHP from the official website (php.net) or use the PHP binaries provided by your chosen web server package.3.
Code Editor
: Choose a code editor or Integrated Development Environment (IDE) for writing PHP code. Some popular options include Visual Studio Code, Sublime Text, PHPStorm, and Atom.Basic PHP Syntax
```php
// PHP code goes here
echo "Hello, World!";
?>
```
PHP code is enclosed within `` tags.
Statements end with a semicolon `;`.
`echo` is used to output text or variables.
Variables and Data Types
```php
$name = "John";
$age = 25;
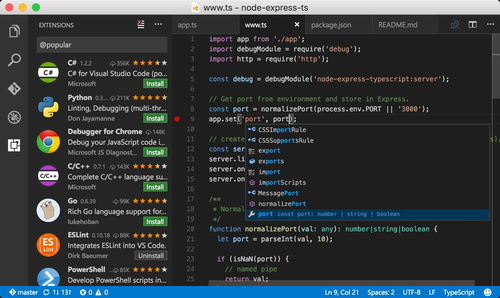
$height = 6.1;
$isMale = true;
?>
```
Variables in PHP start with the dollar sign `$`.
PHP supports various data types, including strings, integers, floats, booleans, arrays, and objects.
Control Structures
```php
// Conditional Statements
if ($age >= 18) {
echo "You are an adult.";
} else {
echo "You are a minor.";
}
// Loops
for ($i = 0; $i < 5; $i ) {
echo $i;
}
// foreach loop
$colors = array("Red", "Green", "Blue");
foreach ($colors as $color) {
echo $color;
}
?>
```
PHP supports common control structures like `if`, `else`, `for`, `while`, and `foreach`.
Functions
```php
function greet($name) {
echo "Hello, $name!";
}
greet("Alice");
?>
```
Functions in PHP are defined using the `function` keyword.
They can accept parameters and return values.
Working with Forms and Databases
PHP is often used to handle form submissions and interact with databases to store and retrieve information.
Handling Forms
```php
$username = $_POST['username'];
$password = $_POST['password'];
// Process form data...
?>
```
Database Interaction (MySQLi)
```php
// Connect to MySQL
$conn = mysqli_connect("localhost", "username", "password", "database");
// Check connection
if (!$conn) {
die("Connection failed: " . mysqli_connect_error());
}
// Perform SQL query
$sql = "SELECT id, name, email FROM users";
$result = mysqli_query($conn, $sql);
// Fetch and display data
if (mysqli_num_rows($result) > 0) {
while ($row = mysqli_fetch_assoc($result)) {
echo "ID: " . $row['id'] . " Name: " . $row['name'] . " Email: " . $row['email'] . "
";
}
} else {
echo "0 results";
}
// Close connection
mysqli_close($conn);
?>
```
Best Practices
Security
: Always validate and sanitize user input to prevent SQL injection and crosssite scripting (XSS) attacks.
Code Organization
: Keep your code modular and organized using functions, classes, and include files.
Error Handling
: Implement error handling and logging to troubleshoot issues.
Performance
: Optimize your code and database queries for better performance.Conclusion
PHP is a versatile language for building dynamic web applications. By understanding its syntax, core concepts, and best practices, you can start developing featurerich websites and web applications. Remember to practice regularly, explore PHP's extensive documentation, and leverage online resources and communities for further learning and support. Happy coding!
Tags: 游戏手柄怎么用 爱心照片拼图 原神飞行考试 火柴人打羽毛球2
版权声明: 免责声明:本网站部分内容由用户自行上传,若侵犯了您的权益,请联系我们处理,谢谢!联系QQ:2760375052
最近发表
- 一款值得信赖的全能座驾
- Jeep牧马人,越野传奇的全面解析
- 轻松掌握 XP 中文语言包下载与安装全攻略
- 深入探索Google操作系统,如何改变我们的数字生活
- 一款独特的美式SUV
- 轻松入门电脑知识,畅游数字世界——电脑知识学习网带你全面掌握
- 深入解读vivo Y93手机参数,性能、功能与用户体验
- 电源已接通但未充电?别慌!详解及解决方法
- 苹果SE4上市时间及价格全解析,性价比之王的回归
- 探寻AM3平台的最佳CPU选择
- 别克君威价格全解析,购车必备指南
- 全面解析与深度评测
- 理解负指数分布图像,隐藏在日常生活中的数学之美
- 全面解析与购车指南
- 深入了解标志206最新报价,购车指南与市场分析
- 深入了解 i3 10100,一款适合日常生活的高效处理器
- 走进vivo手机商城,探索智能生活的新篇章
- 5万以下汽车报价大全,为您精选高性价比的经济型车型
- 一辆小车的精彩故事
- 全面解析与购车建议
- 深入了解昊锐1.8T油耗表现及其优化技巧
- 迈腾18T,都市出行的理想伙伴,轻松驾驭每一段旅程
- 桑塔纳新款,传承经典,焕发新生
- 联发科MT6765,智能手机的高效心脏
- 丰田Previa,一款经典MPV的前世今生
- 小学校长受贿近千万,背后的故事与启示
- 探索移动帝国论坛,连接全球移动技术爱好者的桥梁
- 小小的我预售破4000万,一场梦幻童话的奇迹之旅
- 深度解析凯迪拉克CTS(进口),豪华与性能的完美结合
- 揭秘南方人为何更易患鼻咽癌?
- 豪华与性能的完美结合——价格详解及购车指南
- 我是刑警编剧专访,坚持创作初心,不惯市场之风
- 轻松掌握图标文件的奥秘
- 黄圣依在最强大脑中的高知魅力——路透背后的故事
- 微信紧急提醒,警惕木马病毒——如何防范与应对网络攻击?
- Jeep新大切诺基,经典与现代的完美融合
- 顾客用餐时打火机不慎落入锅内引发爆炸事件解析
- 解读大捷龙报价,购车前必知的关键信息
- 大学生作业中的AI气息,新时代的学习变革
- 比亚迪思锐,探索未来汽车科技的先锋
- 警惕串联他人越级走访,数人多次煽动行为终被抓获的警示
- 经典与现代的完美融合——联想ThinkPad X201,一款改变工作方式的笔记本电脑
- 北京平谷再现鸟中老虎
- 一位七旬官员的人生转折,公诉背后的故事与深思
- 财神鱼离奇死亡,男子悲痛之余做出惊人决定,起锅烧油含泪吃下
- 掌握 Flash 课件制作,从零开始的实用教程
- 蜜雪冰城的新动作,背后的战略调整与市场应对
- 警惕网络谣言,重庆小女孩急需救助的真相揭秘
- 深入了解2012款锋范,经典小车的完美演绎
- 刘诗诗,淡然面对传闻,专注自我成长