您所在的位置:首页 - 科普 - 正文科普
dpdk例子
嵘宸
05-14
【科普】
68人已围观
摘要**Title:GettingStartedwithDPDKProgramming:APracticalExample**---**IntroductiontoDPDK**TheDataPlaneDe
Title: Getting Started with DPDK Programming: A Practical Example
Introduction to DPDK
The Data Plane Development Kit (DPDK) is a set of libraries and drivers for fast packet processing. It provides a framework and set of libraries for fast packet processing in user space. DPDK is widely used in highperformance network applications where lowlatency, highthroughput packet processing is crucial. In this tutorial, we'll walk through a simple DPDK programming example to illustrate its usage and demonstrate how to get started with DPDK development.
Prerequisites
Before diving into DPDK programming, ensure you have the following prerequisites:
1. A Linuxbased system (DPDK primarily supports Linux)
2. Intelcompatible NIC (Network Interface Card) supported by DPDK
3. DPDK library installed on your system
4. Basic knowledge of C programming
Setting Up DPDK Environment
First, make sure you have DPDK installed on your system. You can download the latest version from the DPDK website (https://www.dpdk.org/download/). Follow the installation instructions provided in the DPDK documentation to set up DPDK on your system.
Once DPDK is installed, set up the environment by exporting the required environment variables. These variables typically include `RTE_SDK` (path to DPDK installation directory) and `RTE_TARGET` (target architecture). Here's an example of how you can set up the environment:
```bash
export RTE_SDK=/path/to/your/dpdk/directory
export RTE_TARGET=x86_64nativelinuxappgcc
```
Make sure to adjust the paths according to your DPDK installation directory.
DPDK Programming Example
Now, let's dive into a simple DPDK programming example. We'll create a basic application that initializes DPDK, sets up a network interface, and receives packets from the NIC.
```c
include
include
include
include
include
include
include
include
define RX_RING_SIZE 1024
define NUM_MBUFS 8191
define MBUF_CACHE_SIZE 250
define BURST_SIZE 32
static const struct rte_eth_conf port_conf_default = {
.rxmode = { .max_rx_pkt_len = RTE_ETHER_MAX_LEN }
};
static void dpdk_init(void) {
int ret;
ret = rte_eal_init(argc, argv);
if (ret < 0)
rte_exit(EXIT_FAILURE, "Error initializing DPDK\n");
}
static void dpdk_configure(uint16_t port) {
int ret;
struct rte_eth_conf port_conf = port_conf_default;
const uint16_t rx_rings = 1;
uint16_t nb_rxd = RX_RING_SIZE;
ret = rte_eth_dev_configure(port, rx_rings, 0, &port_conf);
if (ret < 0)
rte_exit(EXIT_FAILURE, "Error configuring port %u\n", port);
ret = rte_eth_rx_queue_setup(port, 0, nb_rxd,
rte_eth_dev_socket_id(port), NULL, NULL);
if (ret < 0)
rte_exit(EXIT_FAILURE, "Error setting up RX queues for port %u\n", port);
ret = rte_eth_dev_start(port);
if (ret < 0)
rte_exit(EXIT_FAILURE, "Error starting port %u\n", port);
}
static void dpdk_receive_packets(uint16_t port) {
struct rte_mbuf *bufs[BURST_SIZE];
int nb_rx;
uint16_t i;
while (1) {
nb_rx = rte_eth_rx_burst(port, 0, bufs, BURST_SIZE);
for (i = 0; i < nb_rx; i ) {
// Process received packets
rte_pktmbuf_free(bufs[i]);
}
}
}
int main(int argc, char **argv) {
uint16_t port_id;
dpdk_init();
port_id = 0; // Change this according to your environment
dpdk_configure(port_id);
dpdk_receive_packets(port_id);
return 0;
}
```
Understanding the Example
dpdk_init():
Initializes DPDK environment.
dpdk_configure():
Configures the specified Ethernet port for packet reception.
dpdk_receive_packets():
Receives packets from the specified port and processes them.Compiling and Running
To compile the example, use the DPDKprovided build system (usually a Makefile). Make sure to link against the DPDK libraries. Once compiled, run the application as sudo (since DPDK requires root privileges) and specify the appropriate commandline arguments.
Conclusion
This example provides a basic understanding of DPDK programming and how to set up a simple packet receiving application using DPDK. Experiment with different DPDK functions and configurations to further explore its capabilities and integrate it into your network applications.
References:
1. DPDK Documentation: https://doc.dpdk.org/guides/
2. DPDK Tutorials: https://core.dpdk.org/doc/tutorials/
3. DPDK Sample Applications: https://core.dpdk.org/doc/sample_app_ug/
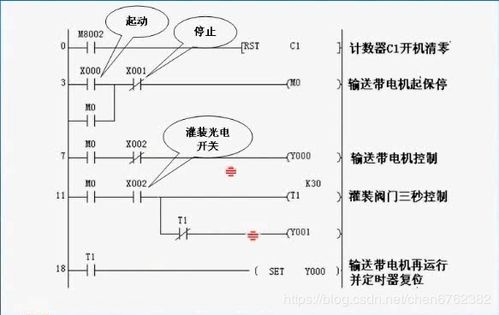
4. Intel Developer Zone DPDK: https://software.intel.com/content/www/us/en/develop/topics/networkingandio/dpdk.html
Tags: 新绝代双骄1 天涯明月刀答题 帮mm脱内衣 真人快打x
版权声明: 免责声明:本网站部分内容由用户自行上传,若侵犯了您的权益,请联系我们处理,谢谢!联系QQ:2760375052
最近发表
- 一款值得信赖的全能座驾
- Jeep牧马人,越野传奇的全面解析
- 轻松掌握 XP 中文语言包下载与安装全攻略
- 深入探索Google操作系统,如何改变我们的数字生活
- 一款独特的美式SUV
- 轻松入门电脑知识,畅游数字世界——电脑知识学习网带你全面掌握
- 深入解读vivo Y93手机参数,性能、功能与用户体验
- 电源已接通但未充电?别慌!详解及解决方法
- 苹果SE4上市时间及价格全解析,性价比之王的回归
- 探寻AM3平台的最佳CPU选择
- 别克君威价格全解析,购车必备指南
- 全面解析与深度评测
- 理解负指数分布图像,隐藏在日常生活中的数学之美
- 全面解析与购车指南
- 深入了解标志206最新报价,购车指南与市场分析
- 深入了解 i3 10100,一款适合日常生活的高效处理器
- 走进vivo手机商城,探索智能生活的新篇章
- 5万以下汽车报价大全,为您精选高性价比的经济型车型
- 一辆小车的精彩故事
- 全面解析与购车建议
- 深入了解昊锐1.8T油耗表现及其优化技巧
- 迈腾18T,都市出行的理想伙伴,轻松驾驭每一段旅程
- 桑塔纳新款,传承经典,焕发新生
- 联发科MT6765,智能手机的高效心脏
- 丰田Previa,一款经典MPV的前世今生
- 小学校长受贿近千万,背后的故事与启示
- 探索移动帝国论坛,连接全球移动技术爱好者的桥梁
- 小小的我预售破4000万,一场梦幻童话的奇迹之旅
- 深度解析凯迪拉克CTS(进口),豪华与性能的完美结合
- 揭秘南方人为何更易患鼻咽癌?
- 豪华与性能的完美结合——价格详解及购车指南
- 我是刑警编剧专访,坚持创作初心,不惯市场之风
- 轻松掌握图标文件的奥秘
- 黄圣依在最强大脑中的高知魅力——路透背后的故事
- 微信紧急提醒,警惕木马病毒——如何防范与应对网络攻击?
- Jeep新大切诺基,经典与现代的完美融合
- 顾客用餐时打火机不慎落入锅内引发爆炸事件解析
- 解读大捷龙报价,购车前必知的关键信息
- 大学生作业中的AI气息,新时代的学习变革
- 比亚迪思锐,探索未来汽车科技的先锋
- 警惕串联他人越级走访,数人多次煽动行为终被抓获的警示
- 经典与现代的完美融合——联想ThinkPad X201,一款改变工作方式的笔记本电脑
- 北京平谷再现鸟中老虎
- 一位七旬官员的人生转折,公诉背后的故事与深思
- 财神鱼离奇死亡,男子悲痛之余做出惊人决定,起锅烧油含泪吃下
- 掌握 Flash 课件制作,从零开始的实用教程
- 蜜雪冰城的新动作,背后的战略调整与市场应对
- 警惕网络谣言,重庆小女孩急需救助的真相揭秘
- 深入了解2012款锋范,经典小车的完美演绎
- 刘诗诗,淡然面对传闻,专注自我成长