您所在的位置:首页 - 百科 - 正文百科
stm8用什么软件编程
晟茼
2024-05-14
【百科】
587人已围观
摘要**Title:BasicCProgrammingforSTM8Series**BasicCProgrammingforSTM8SeriesBasicCProgrammingforSTM8Series
Title: Basic C Programming for STM8 Series
Basic C Programming for STM8 Series
The STM8 series microcontrollers are widely used in various embedded systems applications due to their low cost, low power consumption, and rich peripheral set. Programming these microcontrollers in C language provides an efficient way to utilize their capabilities. Below are the fundamentals of C programming for STM8 series microcontrollers:
Before starting with programming, set up the development environment:
- Download and install the STM8CubeMX software for configuration and code generation.
- Install the SDCC (Small Device C Compiler) toolchain for compiling C code.
- Select the appropriate STM8 microcontroller and configure the clock, peripherals, and pins using STM8CubeMX.
GPIO pins are used for digital input and output operations. Here's how to configure and use GPIOs:
- Configure GPIO pins as input or output using the GPIO_Init() function.
- Read the input state using GPIO_ReadInputDataBit() function.
- Write output state using GPIO_Write() function.
Interrupts are essential for handling asynchronous events. Here's how to handle interrupts:
- Enable global interrupts using the enable_interrupts() function.
- Configure specific interrupt sources and their priorities.
- Write interrupt service routines (ISRs) to handle the interrupt events.
Timers and PWM are crucial for generating precise time delays and controlling motor speeds, brightness, etc.:
- Initialize timers using TIM4_Init() function.
- Configure timer modes (e.g., PWM mode) and prescaler values.
- Generate PWM signals using TIM4_SetCompare() function.
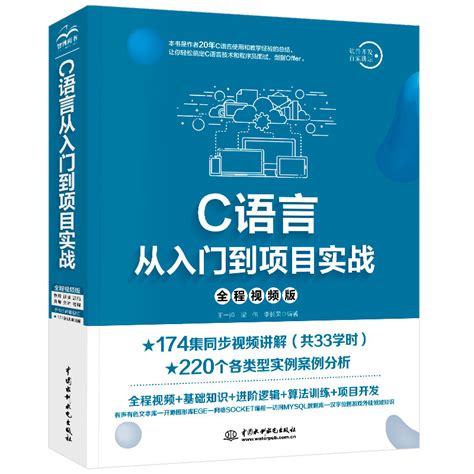
ADC is used to convert analog signals to digital values for processing:
- Configure ADC channels using ADC1_Init() function.
- Start ADC conversion using ADC1_GetConversionValue() function.
- Handle conversion completion interrupts and process the digital values.
UART is used for serial communication with other devices:
- Initialize UART communication using UART1_Init() function.
- Configure baud rate, data bits, stop bits, and parity.
- Transmit and receive data using UART1_Write() and UART1_Read() functions.
STM8 microcontrollers have limited RAM and ROM, so efficient memory management is essential:
- Use const qualifier for readonly data stored in ROM.
- Optimize variable usage to conserve RAM.
- Avoid dynamic memory allocation.
Debugging tools are crucial for identifying and fixing issues:
- Use printf() function for debugging via UART.
- Utilize breakpoints and watch variables in the debugger.
- Perform thorough testing of code on hardware.
Mastering C programming for STM8 series microcontrollers requires a solid understanding of fundamental concepts such as GPIO, interrupts, timers, communication protocols, and memory management. By following the guidelines and best practices outlined above, developers can efficiently utilize the capabilities of STM8 microcontrollers in embedded systems applications.