您所在的位置:首页 - 热点 - 正文热点
单片机c程序设计100例
进宝
05-10
【热点】
181人已围观
摘要**Title:PracticalExamplesofCProgrammingforMicrocontrollers**ProgrammingmicrocontrollersinCoffersapow
Title: Practical Examples of C Programming for Microcontrollers
Programming microcontrollers in C offers a powerful platform for embedded systems development. Below are some practical examples showcasing various functionalities and concepts in C programming for microcontrollers.
Example 1: Blinking LED
```c
include
include
define LED_PIN PB0 // Define LED pin
int main(void) {
DDRB |= (1 << LED_PIN); // Set LED pin as output
while (1) {
PORTB ^= (1 << LED_PIN); // Toggle LED state
_delay_ms(500); // Delay for 500ms
}
return 0;
}
```
This example demonstrates the basic "Hello World" program for microcontrollers by blinking an LED connected to pin PB0.
Example 2: Reading Analog Sensor
```c
include
include
define SENSOR_PIN PC0 // Define sensor pin
void ADC_init() {
// Set reference voltage and enable ADC
ADMUX |= (1 << REFS0);
ADCSRA |= (1 << ADEN);
}
uint16_t ADC_read(uint8_t channel) {
ADMUX = (ADMUX & 0xF0) | (channel & 0x0F); // Select ADC channel
ADCSRA |= (1 << ADSC); // Start conversion
while (ADCSRA & (1 << ADSC)); // Wait for conversion to complete
return ADC;
}
int main(void) {
uint16_t sensorValue;
ADC_init();
while (1) {
sensorValue = ADC_read(SENSOR_PIN);
// Process sensor value
_delay_ms(1000); // Delay for 1 second
}
return 0;
}
```
This example reads an analog sensor connected to pin PC0 using the builtin ADC of the microcontroller.
Example 3: UART Communication
```c
include
include
define F_CPU 16000000UL // Define CPU frequency
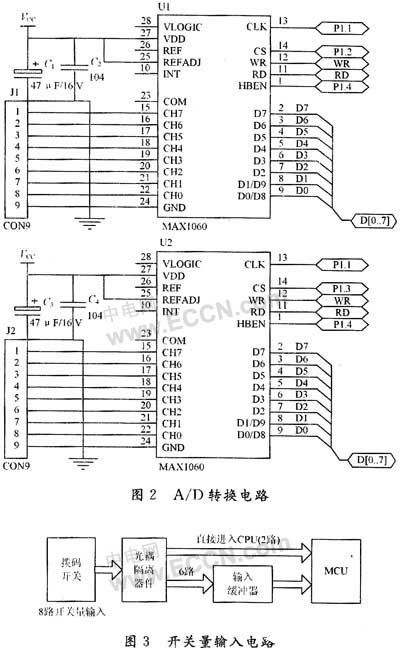
define BAUDRATE 9600
define UBRR_VAL ((F_CPU / (BAUDRATE * 16UL)) 1)
void UART_init() {
UBRR0H = (uint8_t)(UBRR_VAL >> 8); // Set baud rate
UBRR0L = (uint8_t)UBRR_VAL;
UCSR0B = (1 << TXEN0) | (1 << RXEN0); // Enable transmitter and receiver
UCSR0C = (1 << UCSZ01) | (1 << UCSZ00); // Set frame format: 8 data bits, 1 stop bit
}
void UART_transmit(uint8_t data) {
while (!(UCSR0A & (1 << UDRE0))); // Wait for empty transmit buffer
UDR0 = data; // Put data into buffer, sends the data
}
uint8_t UART_receive() {
while (!(UCSR0A & (1 << RXC0))); // Wait for data to be received
return UDR0; // Get and return received data from buffer
}
int main(void) {
uint8_t receivedData;
UART_init();
while (1) {
receivedData = UART_receive();
// Process received data
UART_transmit(receivedData); // Echo back received data
}
return 0;
}
```
This example demonstrates UART communication for serial data transmission and reception.
Example 4: PWM Control
```c
include
include
define PWM_PIN PB1 // Define PWM pin
void PWM_init() {
TCCR1A |= (1 << COM1A1) | (1 << WGM11); // Set noninverting mode and fast PWM mode
TCCR1B |= (1 << WGM12) | (1 << WGM13) | (1 << CS11); // Set prescaler to 8
OCR1A = 128; // Set initial duty cycle
DDRB |= (1 << PWM_PIN); // Set PWM pin as output
}
int main(void) {
PWM_init();
while (1) {
// Adjust duty cycle for desired brightness or motor speed
_delay_ms(1000); // Delay for 1 second
}
return 0;
}
```
This example demonstrates pulse width modulation (PWM) for controlling the brightness of an LED or speed of a motor.
These examples cover fundamental aspects of C programming for microcontrollers, including GPIO manipulation, analog input, serial communication, and PWM control. Experimenting with these examples will provide a solid foundation for further exploration in embedded systems development.
Tags: 三岁激活码生成器 梦幻西游乌鸡国副本 原神钓鱼点 无中介租房 铁血狙击手
版权声明: 免责声明:本网站部分内容由用户自行上传,若侵犯了您的权益,请联系我们处理,谢谢!联系QQ:2760375052
最近发表
- 三星手机的用户群像,谁在用,为什么选择它?
- 上海通用雪佛兰景程——家用轿车的理想选择
- 雪佛兰新赛欧油耗深度解析,经济实惠的出行选择
- 一款值得信赖的全能座驾
- Jeep牧马人,越野传奇的全面解析
- 轻松掌握 XP 中文语言包下载与安装全攻略
- 深入探索Google操作系统,如何改变我们的数字生活
- 一款独特的美式SUV
- 轻松入门电脑知识,畅游数字世界——电脑知识学习网带你全面掌握
- 深入解读vivo Y93手机参数,性能、功能与用户体验
- 电源已接通但未充电?别慌!详解及解决方法
- 苹果SE4上市时间及价格全解析,性价比之王的回归
- 探寻AM3平台的最佳CPU选择
- 别克君威价格全解析,购车必备指南
- 全面解析与深度评测
- 理解负指数分布图像,隐藏在日常生活中的数学之美
- 全面解析与购车指南
- 深入了解标志206最新报价,购车指南与市场分析
- 深入了解 i3 10100,一款适合日常生活的高效处理器
- 走进vivo手机商城,探索智能生活的新篇章
- 5万以下汽车报价大全,为您精选高性价比的经济型车型
- 一辆小车的精彩故事
- 全面解析与购车建议
- 深入了解昊锐1.8T油耗表现及其优化技巧
- 迈腾18T,都市出行的理想伙伴,轻松驾驭每一段旅程
- 桑塔纳新款,传承经典,焕发新生
- 联发科MT6765,智能手机的高效心脏
- 丰田Previa,一款经典MPV的前世今生
- 小学校长受贿近千万,背后的故事与启示
- 探索移动帝国论坛,连接全球移动技术爱好者的桥梁
- 小小的我预售破4000万,一场梦幻童话的奇迹之旅
- 深度解析凯迪拉克CTS(进口),豪华与性能的完美结合
- 揭秘南方人为何更易患鼻咽癌?
- 豪华与性能的完美结合——价格详解及购车指南
- 我是刑警编剧专访,坚持创作初心,不惯市场之风
- 轻松掌握图标文件的奥秘
- 黄圣依在最强大脑中的高知魅力——路透背后的故事
- 微信紧急提醒,警惕木马病毒——如何防范与应对网络攻击?
- Jeep新大切诺基,经典与现代的完美融合
- 顾客用餐时打火机不慎落入锅内引发爆炸事件解析
- 解读大捷龙报价,购车前必知的关键信息
- 大学生作业中的AI气息,新时代的学习变革
- 比亚迪思锐,探索未来汽车科技的先锋
- 警惕串联他人越级走访,数人多次煽动行为终被抓获的警示
- 经典与现代的完美融合——联想ThinkPad X201,一款改变工作方式的笔记本电脑
- 北京平谷再现鸟中老虎
- 一位七旬官员的人生转折,公诉背后的故事与深思
- 财神鱼离奇死亡,男子悲痛之余做出惊人决定,起锅烧油含泪吃下
- 掌握 Flash 课件制作,从零开始的实用教程
- 蜜雪冰城的新动作,背后的战略调整与市场应对