您所在的位置:首页 - 生活 - 正文生活
把多个变量变成一个变量
小见
2024-05-04
【生活】
62人已围观
摘要**Title:ManagingMultipleVariablesinProgramming**Inprogramming,handlingmultiplevariablesefficientlyis
Title: Managing Multiple Variables in Programming
In programming, handling multiple variables efficiently is crucial for writing clean, maintainable, and scalable code. Whether you're working on a small script or a largescale application, effective management of variables can significantly impact the performance and readability of your code. Let's delve into some key practices and strategies for managing multiple variables in programming:
1. Use Descriptive Variable Names:
Choosing descriptive and meaningful variable names can enhance code readability and maintainability. Instead of singleletter or ambiguous names, opt for names that clearly convey the purpose or content of the variable.
```python
Bad example
a = 10
b = 20
Good example
total_students = 10
passing_score = 20
```
2. Group Related Variables:
Grouping related variables together can improve code organization and comprehension. You can use data structures like dictionaries or classes to encapsulate related variables.
```python
Using dictionaries
student = {
"name": "John",
"age": 20,
"grade": "A"
}
Using classes
class Student:
def __init__(self, name, age, grade):
self.name = name
self.age = age
self.grade = grade
john = Student("John", 20, "A")
```
3. Avoid Global Variables:
Minimize the use of global variables as they can lead to tight coupling and make code harder to debug and test. Instead, prefer passing variables as parameters to functions or encapsulating them within classes.
```python
Avoid global variables
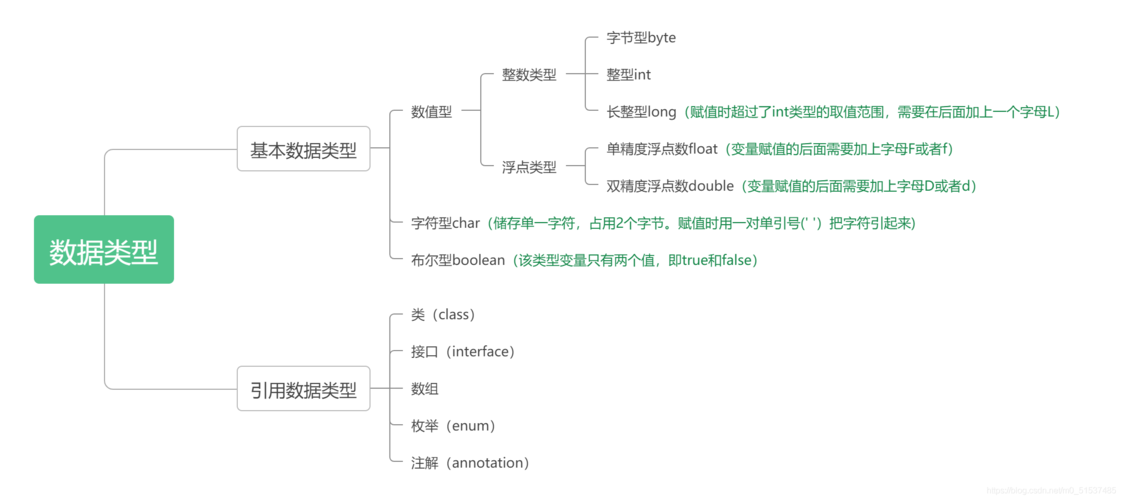
total_students = 100
def calculate_percentage():
global total_students
return (total_students / 1000) * 100
Prefer passing variables as parameters
def calculate_percentage(total_students):
return (total_students / 1000) * 100
```
4. Distinguish Between Constants and Variables:
Clearly differentiate between constants (unchanging values) and variables (changing values) in your code. Use uppercase names for constants to indicate that their values should not be modified.
```python
Constants
PI = 3.14
MAX_SIZE = 100
Variables
radius = 5
```
5. Avoid Magic Numbers:
Avoid using magic numbers (hardcoded numerical values) in your code. Instead, assign them to named variables or constants to improve code readability and maintainability.
```python
Magic number
area = 3.14 * radius * radius
Named variable
pi = 3.14
area = pi * radius * radius
```
6. Use Data Structures Wisely:
Choose appropriate data structures based on the requirements of your program. Lists, tuples, dictionaries, and sets offer different capabilities, so select the one that best fits your use case.
```python
Using lists
grades = [80, 90, 75, 85]
Using dictionaries
student_grades = {"John": 80, "Alice": 90, "Bob": 75, "Emma": 85}
```
Conclusion:
Effectively managing multiple variables in programming involves employing good naming conventions, organizing related variables, minimizing global usage, distinguishing between constants and variables, avoiding magic numbers, and selecting appropriate data structures. By following these practices, you can write cleaner, more maintainable code that is easier to understand and debug.
Tags: 定时关机3000破解版 永不消失的彩虹 热血龙城手游 与exo的校园生活
版权声明: 免责声明:本网站部分内容由用户自行上传,若侵犯了您的权益,请联系我们处理,谢谢!联系QQ:2760375052
上一篇: 核桃编程课
下一篇: 鲸鱼编程机器人教育版
最近发表
- 特朗普回应普京涉乌言论,强硬立场引发争议与担忧
- 民营企业如何向新而行——探索创新发展的路径与实践
- 联合国秘书长视角下的普京提议,深度解析与理解
- 广东茂名发生地震,一次轻微震动带来的启示与思考
- 刀郎演唱会外,上千歌迷的守候与共鸣
- 东北夫妻开店遭遇刁难?当地回应来了
- 特朗普惊人言论,为夺取格陵兰岛,美国不排除动用武力
- 超级食物在中国,掀起健康热潮
- 父爱无声胜有声,监控摄像头背后的温情呼唤
- 泥坑中的拥抱,一次意外的冒险之旅
- 成品油需求变天,市场趋势下的新机遇与挑战
- 警惕儿童健康隐患,10岁女孩因高烧去世背后的警示
- 提振消费,新举措助力消费复苏
- 蒙牛净利润暴跌98%的背后原因及未来展望
- 揭秘缅甸强震背后的真相,并非意外事件
- 揭秘失踪的清华毕业生罗生门背后的悲剧真相
- 冷空气终于要走了,春天的脚步近了
- 李乃文的神奇之笔,与和伟的奇妙转变
- 妹妹发现植物人哥哥离世后的崩溃大哭,生命的脆弱与情感的冲击
- 云南曲靖市会泽县发生4.4级地震,深入了解与应对之道
- 缅甸政府部门大楼倒塌事件,多名官员伤亡,揭示背后的故事
- 多方合力寻找失踪的十二岁少女,七天生死大搜寻
- S妈情绪崩溃,小S拒绝好友聚会背后的故事
- 缅甸遭遇地震,灾难之下的人间故事与影响深度解析
- 缅甸地震与瑞丽市中心高楼砖石坠落事件揭秘
- 揭秘ASP集中营,技术成长的摇篮与挑战
- 徐彬,整场高位压迫对海港形成巨大压力——战术分析与实践洞察
- ThreadX操作系统,轻量、高效与未来的嵌入式开发新选择
- 王钰栋脚踝被踩事件回应,伤势并不严重,一切都在恢复中
- 刘亦菲,粉色花瓣裙美神降临
- 三星W2018与G9298,高端翻盖手机的对比分析
- 多哈世乒赛器材,赛场内外的热议焦点
- K2两厢车,小巧灵活的城市出行神器,适合你的生活吗?
- 国家市监局将审查李嘉诚港口交易,聚焦市场关注焦点
- 提升知识水平的趣味之旅
- 清明五一档电影市场繁荣,多部影片争相上映,你期待哪一部?
- 美联储再次面临痛苦抉择,权衡通胀与经济恢复
- 家庭千万别买投影仪——真相大揭秘!
- 文物当上网红后,年轻人的创意与传承之道
- 手机解除Root的最简单方法,安全、快速、易操作
- 缅甸地震与汶川地震,能量的震撼与对比
- 2011款奥迪A8,豪华与科技的完美结合
- 广州惊艳亮相,可折叠电动垂直起降飞行器革新城市交通方式
- 比亚迪F3最低报价解析,性价比之选的购车指南
- 商业健康保险药品征求意见,行业内外视角与实用建议
- 官方动态解读,最低工资标准的合理调整
- 东风标致5008最新报价出炉,性价比杀手来了!
- 大陆配偶在台湾遭遇限期离台风波,各界发声背后的故事与影响
- 奔驰C级2022新款,豪华与科技的完美融合
- 大摩小摩去年四季度对A股的投资热潮