您所在的位置:首页 - 科普 - 正文科普
UDP编程与TCP编程有什么区别
太荣
05-04
【科普】
133人已围观
摘要**Title:UDPProgramminginPython:AComprehensiveGuide**UDP(UserDatagramProtocol)isaconnectionlessandlig
Title: UDP Programming in Python: A Comprehensive Guide
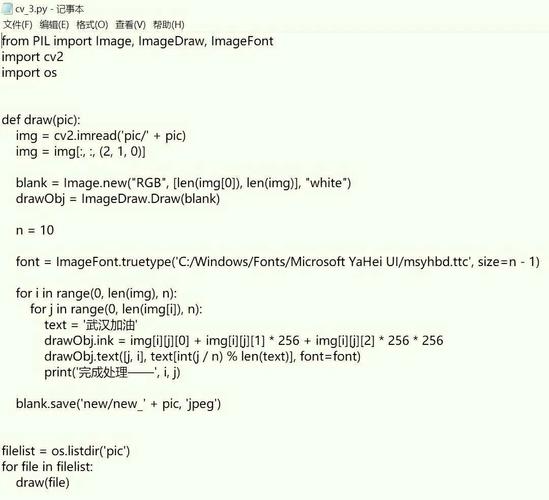
UDP (User Datagram Protocol) is a connectionless and lightweight transport protocol widely used in networking applications where efficiency and low overhead are crucial. Python provides robust support for UDP programming through its builtin `socket` module. This guide will walk you through the fundamentals of UDP programming in Python, covering socket creation, sending and receiving data, error handling, and best practices.
Getting Started with UDP Socket Creation
To start UDP programming in Python, you need to create a UDP socket using the `socket` module. Here's how you can do it:
```python
import socket
Create a UDP socket
udp_socket = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
```
In the `socket.socket()` function:
`AF_INET` indicates the address family, which in this case is IPv4.
`SOCK_DGRAM` specifies the socket type as UDP.
Sending Data over UDP
Once you've created the UDP socket, you can send data to a specific destination using the `sendto()` method:
```python
Send data over UDP
message = "Hello, UDP!"
dest_address = ('127.0.0.1', 12345) Example destination IP and port
udp_socket.sendto(message.encode(), dest_address)
```
In the `sendto()` method:
The first argument is the data to be sent, encoded as bytes.
The second argument is a tuple containing the destination IP address and port number.
Receiving Data over UDP
Receiving data in UDP involves calling the `recvfrom()` method:
```python
Receive data over UDP
buffer_size = 1024
data, source_address = udp_socket.recvfrom(buffer_size)
print("Received:", data.decode())
print("From:", source_address)
```
In the `recvfrom()` method:
The argument specifies the maximum amount of data to be received at once.
It returns a tuple containing the received data and the address of the sender.
Closing the UDP Socket
Don't forget to close the UDP socket once you're done using it:
```python
Close the UDP socket
udp_socket.close()
```
Error Handling
Error handling is essential in UDP programming to deal with potential issues such as network errors or incorrect configurations. Here's an example of how to handle errors:
```python
try:
UDP socket operations
except socket.error as e:
print("Socket error:", e)
finally:
udp_socket.close()
```
Best Practices and Recommendations
1.
Use Reliable Error Handling
: Always wrap UDP socket operations in tryexcept blocks to handle errors gracefully.2.
Implement Timeout Mechanisms
: UDP is unreliable and does not guarantee packet delivery. Implement timeout mechanisms to handle lost or delayed packets.3.
Consider Packet Loss and Order
: UDP does not ensure packet delivery order or reliability. If your application requires reliability, consider using TCP instead.4.
Optimize Data Size
: Keep UDP datagrams small to minimize the risk of fragmentation and improve efficiency.5.
Security Considerations
: Implement additional security measures if transmitting sensitive data over UDP, as it does not provide builtin encryption or authentication.Conclusion
UDP programming in Python using the `socket` module offers a powerful and flexible way to build networked applications. By understanding the fundamentals of UDP socket creation, data transmission, error handling, and best practices, you can develop efficient and reliable UDPbased systems tailored to your specific requirements.
Now that you have a solid understanding of UDP programming in Python, feel free to explore more advanced topics such as multicast communication, asynchronous UDP, or integrating UDP with other network protocols. Happy coding!
Note:
Remember to always refer to the Python documentation for the most uptodate information and additional examples.Tags: 剑网三超级宏 手机网络游戏 旗帜软件照片处理工具 勇气点数上限
版权声明: 免责声明:本网站部分内容由用户自行上传,若侵犯了您的权益,请联系我们处理,谢谢!联系QQ:2760375052
最近发表
- Jeep牧马人,越野传奇的全面解析
- 轻松掌握 XP 中文语言包下载与安装全攻略
- 深入探索Google操作系统,如何改变我们的数字生活
- 一款独特的美式SUV
- 轻松入门电脑知识,畅游数字世界——电脑知识学习网带你全面掌握
- 深入解读vivo Y93手机参数,性能、功能与用户体验
- 电源已接通但未充电?别慌!详解及解决方法
- 苹果SE4上市时间及价格全解析,性价比之王的回归
- 探寻AM3平台的最佳CPU选择
- 别克君威价格全解析,购车必备指南
- 全面解析与深度评测
- 理解负指数分布图像,隐藏在日常生活中的数学之美
- 全面解析与购车指南
- 深入了解标志206最新报价,购车指南与市场分析
- 深入了解 i3 10100,一款适合日常生活的高效处理器
- 走进vivo手机商城,探索智能生活的新篇章
- 5万以下汽车报价大全,为您精选高性价比的经济型车型
- 一辆小车的精彩故事
- 全面解析与购车建议
- 深入了解昊锐1.8T油耗表现及其优化技巧
- 迈腾18T,都市出行的理想伙伴,轻松驾驭每一段旅程
- 桑塔纳新款,传承经典,焕发新生
- 联发科MT6765,智能手机的高效心脏
- 丰田Previa,一款经典MPV的前世今生
- 小学校长受贿近千万,背后的故事与启示
- 探索移动帝国论坛,连接全球移动技术爱好者的桥梁
- 小小的我预售破4000万,一场梦幻童话的奇迹之旅
- 深度解析凯迪拉克CTS(进口),豪华与性能的完美结合
- 揭秘南方人为何更易患鼻咽癌?
- 豪华与性能的完美结合——价格详解及购车指南
- 我是刑警编剧专访,坚持创作初心,不惯市场之风
- 轻松掌握图标文件的奥秘
- 黄圣依在最强大脑中的高知魅力——路透背后的故事
- 微信紧急提醒,警惕木马病毒——如何防范与应对网络攻击?
- Jeep新大切诺基,经典与现代的完美融合
- 顾客用餐时打火机不慎落入锅内引发爆炸事件解析
- 解读大捷龙报价,购车前必知的关键信息
- 大学生作业中的AI气息,新时代的学习变革
- 比亚迪思锐,探索未来汽车科技的先锋
- 警惕串联他人越级走访,数人多次煽动行为终被抓获的警示
- 经典与现代的完美融合——联想ThinkPad X201,一款改变工作方式的笔记本电脑
- 北京平谷再现鸟中老虎
- 一位七旬官员的人生转折,公诉背后的故事与深思
- 财神鱼离奇死亡,男子悲痛之余做出惊人决定,起锅烧油含泪吃下
- 掌握 Flash 课件制作,从零开始的实用教程
- 蜜雪冰城的新动作,背后的战略调整与市场应对
- 警惕网络谣言,重庆小女孩急需救助的真相揭秘
- 深入了解2012款锋范,经典小车的完美演绎
- 刘诗诗,淡然面对传闻,专注自我成长
- 开启搜索引擎优化与数字营销的新旅程