您所在的位置:首页 - 百科 - 正文百科
链表怎么输入数据
蒽博
05-04
【百科】
697人已围观
摘要编程链表是数据结构中的一个重要概念,它可以用于存储和管理大量数据。在这里,我将介绍如何编程实现链表。链表是由一组节点组成的数据结构,每个节点包含数据和一个指向下一个节点的指针。链表有头节点和尾节点,头
编程链表是数据结构中的一个重要概念,它可以用于存储和管理大量数据。在这里,我将介绍如何编程实现链表。
链表是由一组节点组成的数据结构,每个节点包含数据和一个指向下一个节点的指针。链表有头节点和尾节点,头节点保存链表的第一个节点,尾节点保存链表的最后一个节点。
下面是一个简单的链表节点的定义:
```
class Node {
int data;
Node next;
Node(int data) {
this.data = data;
this.next = null;
}
}
```
接下来是一个链表的实现类:
```
class LinkedList {
Node head;
Node tail;
LinkedList() {
this.head = null;
this.tail = null;
}
// 在链表末尾插入一个节点
void append(int data) {
Node newNode = new Node(data);
if (head == null) {
head = newNode;
tail = newNode;
} else {
tail.next = newNode;
tail = newNode;
}
}
// 在链表的指定位置插入一个节点
void insert(int data, int position) {
Node newNode = new Node(data);
if (position == 0) {
newNode.next = head;
head = newNode;
} else {
Node current = head;
int currentPosition = 0;
while (currentPosition < position 1 && current != null) {
current = current.next;
currentPosition ;
}
if (current != null) {
newNode.next = current.next;
current.next = newNode;
}
}
}
// 删除链表指定位置的节点
void delete(int position) {
if (head == null) {
return;
}
if (position == 0) {
head = head.next;
} else {
Node current = head;
int currentPosition = 0;
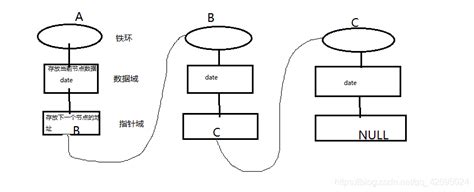
while (currentPosition < position 1 && current != null) {
current = current.next;
currentPosition ;
}
if (current != null && current.next != null) {
current.next = current.next.next;
}
}
}
// 输出链表的内容
void display() {
Node current = head;
while (current != null) {
System.out.print(current.data " ");
current = current.next;
}
}
}
```
以上是一个基本的链表的实现。你可以使用以下代码进行测试:
```
public class Main {
public static void main(String[] args) {
LinkedList linkedList = new LinkedList();
linkedList.append(1);
linkedList.append(2);
linkedList.append(3);
linkedList.insert(4, 1);
linkedList.insert(5, 3);
linkedList.delete(2);
linkedList.display(); // 输出:1 4 5 3
}
}
```
使用以上代码,你可以在Java中实现一个简单的链表数据结构。当然,你还可以根据需要添加其他方法,如查找特定节点、反转链表等。
希望这能帮到你,如果你对链表的其他操作有疑问,可以随时向我提问。
Tags: 谁在背我飞行 江南百景图严大人交换表 艾泽拉斯人口普查 塞纳里奥议会 盗墓迷城2
版权声明: 免责声明:本网站部分内容由用户自行上传,若侵犯了您的权益,请联系我们处理,谢谢!联系QQ:2760375052
最近发表
- 雪佛兰新赛欧油耗深度解析,经济实惠的出行选择
- 一款值得信赖的全能座驾
- Jeep牧马人,越野传奇的全面解析
- 轻松掌握 XP 中文语言包下载与安装全攻略
- 深入探索Google操作系统,如何改变我们的数字生活
- 一款独特的美式SUV
- 轻松入门电脑知识,畅游数字世界——电脑知识学习网带你全面掌握
- 深入解读vivo Y93手机参数,性能、功能与用户体验
- 电源已接通但未充电?别慌!详解及解决方法
- 苹果SE4上市时间及价格全解析,性价比之王的回归
- 探寻AM3平台的最佳CPU选择
- 别克君威价格全解析,购车必备指南
- 全面解析与深度评测
- 理解负指数分布图像,隐藏在日常生活中的数学之美
- 全面解析与购车指南
- 深入了解标志206最新报价,购车指南与市场分析
- 深入了解 i3 10100,一款适合日常生活的高效处理器
- 走进vivo手机商城,探索智能生活的新篇章
- 5万以下汽车报价大全,为您精选高性价比的经济型车型
- 一辆小车的精彩故事
- 全面解析与购车建议
- 深入了解昊锐1.8T油耗表现及其优化技巧
- 迈腾18T,都市出行的理想伙伴,轻松驾驭每一段旅程
- 桑塔纳新款,传承经典,焕发新生
- 联发科MT6765,智能手机的高效心脏
- 丰田Previa,一款经典MPV的前世今生
- 小学校长受贿近千万,背后的故事与启示
- 探索移动帝国论坛,连接全球移动技术爱好者的桥梁
- 小小的我预售破4000万,一场梦幻童话的奇迹之旅
- 深度解析凯迪拉克CTS(进口),豪华与性能的完美结合
- 揭秘南方人为何更易患鼻咽癌?
- 豪华与性能的完美结合——价格详解及购车指南
- 我是刑警编剧专访,坚持创作初心,不惯市场之风
- 轻松掌握图标文件的奥秘
- 黄圣依在最强大脑中的高知魅力——路透背后的故事
- 微信紧急提醒,警惕木马病毒——如何防范与应对网络攻击?
- Jeep新大切诺基,经典与现代的完美融合
- 顾客用餐时打火机不慎落入锅内引发爆炸事件解析
- 解读大捷龙报价,购车前必知的关键信息
- 大学生作业中的AI气息,新时代的学习变革
- 比亚迪思锐,探索未来汽车科技的先锋
- 警惕串联他人越级走访,数人多次煽动行为终被抓获的警示
- 经典与现代的完美融合——联想ThinkPad X201,一款改变工作方式的笔记本电脑
- 北京平谷再现鸟中老虎
- 一位七旬官员的人生转折,公诉背后的故事与深思
- 财神鱼离奇死亡,男子悲痛之余做出惊人决定,起锅烧油含泪吃下
- 掌握 Flash 课件制作,从零开始的实用教程
- 蜜雪冰城的新动作,背后的战略调整与市场应对
- 警惕网络谣言,重庆小女孩急需救助的真相揭秘
- 深入了解2012款锋范,经典小车的完美演绎