您所在的位置:首页 - 百科 - 正文百科
攻丝编程实例
妍锐
05-02
【百科】
138人已围观
摘要**Title:UnderstandingandImplementingThreadinginProgramming**Threading,inthecontextofprogramming,refe
Title: Understanding and Implementing Threading in Programming
Threading, in the context of programming, refers to the ability of a program to execute multiple tasks concurrently. This concept is particularly crucial in scenarios where performance optimization and responsiveness are critical, such as in application development, serverside programming, and computational tasks. In this guide, we'll delve into the fundamentals of threading, its implementation in various programming languages, common challenges, and best practices.
Understanding Threading
1.
What is Threading?
Threading involves the simultaneous execution of multiple tasks within a single process. Each task runs independently, allowing for parallelism and improved performance. Threads share the same memory space, making data sharing and communication between threads relatively easy.
2.
Thread vs. Process
Threads are lighter than processes since they share resources such as memory and file handles. Processes, on the other hand, are standalone entities with their own memory space. Threads within a process can communicate more efficiently than processes, but they also require careful synchronization to avoid issues like race conditions.
3.
Benefits of Threading
Threading offers several advantages, including:
Improved performance by utilizing multiple CPU cores.
Enhanced responsiveness, especially in GUI applications where longrunning tasks can freeze the user interface.
Efficient resource utilization by sharing memory and other resources among threads.
Implementing Threading in Programming Languages
1.
Python: Threading Module
Python provides a builtin `threading` module for implementing threading. However, due to the Global Interpreter Lock (GIL), which allows only one thread to execute Python bytecode at a time, threading in Python is more suitable for I/Obound tasks rather than CPUbound tasks.
```python
import threading
def task():
Your task implementation here
thread = threading.Thread(target=task)
thread.start()
```
2.
Java: java.lang.Thread Class
In Java, threading is implemented using the `Thread` class or by implementing the `Runnable` interface. Java's threading model allows for both CPUbound and I/Obound tasks.
```java
class MyThread extends Thread {
public void run() {
// Your task implementation here
}
}
MyThread thread = new MyThread();
thread.start();
```
3.
C : std::thread Library
C 11 introduced the `
```cpp
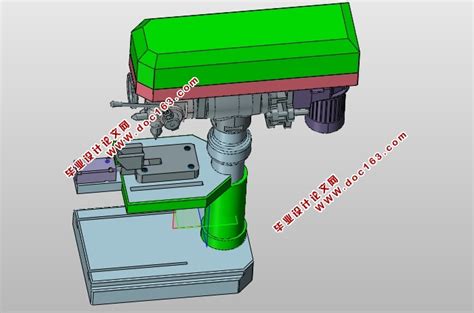
include
void task() {
// Your task implementation here
}
std::thread thread(task);
thread.join();
```
Challenges and Best Practices
1.
Synchronization and Deadlocks
Proper synchronization is essential when multiple threads access shared resources to avoid data corruption or inconsistent states. Techniques such as mutexes, semaphores, and locks are used to synchronize access.
2.
Deadlocks
Deadlocks occur when two or more threads are waiting indefinitely for each other to release resources. Avoiding deadlocks requires careful design and adherence to best practices, such as acquiring locks in a consistent order.
3.
Performance Overhead
Threading introduces overhead due to context switching and synchronization. Profiling tools can help identify performance bottlenecks, and techniques like thread pooling can mitigate overhead by reusing threads.
4.
Use Cases and Considerations
Threading is suitable for tasks that can be divided into smaller, independent units of work. However, not all tasks benefit from threading, and in some cases, the overhead may outweigh the performance gains. Consider factors such as task granularity, resource constraints, and platform limitations when deciding whether to use threading.
Conclusion
Threading is a powerful technique for achieving concurrency and improving the performance and responsiveness of software applications. By understanding the fundamentals of threading, implementing it effectively in various programming languages, and adhering to best practices, developers can harness the full potential of multithreaded programming while avoiding common pitfalls. Threading enables applications to make efficient use of modern multicore processors and deliver a seamless user experience across a wide range of computing environments.
Tags: 孙尚香是谁 个税计算器2020 魔兽世界阿克蒙德 阿加雷斯特战记zero
版权声明: 免责声明:本网站部分内容由用户自行上传,若侵犯了您的权益,请联系我们处理,谢谢!联系QQ:2760375052
上一篇: 乌鸦游戏视频解说
下一篇: 常用的plc编程软件
最近发表
- 一款值得信赖的全能座驾
- Jeep牧马人,越野传奇的全面解析
- 轻松掌握 XP 中文语言包下载与安装全攻略
- 深入探索Google操作系统,如何改变我们的数字生活
- 一款独特的美式SUV
- 轻松入门电脑知识,畅游数字世界——电脑知识学习网带你全面掌握
- 深入解读vivo Y93手机参数,性能、功能与用户体验
- 电源已接通但未充电?别慌!详解及解决方法
- 苹果SE4上市时间及价格全解析,性价比之王的回归
- 探寻AM3平台的最佳CPU选择
- 别克君威价格全解析,购车必备指南
- 全面解析与深度评测
- 理解负指数分布图像,隐藏在日常生活中的数学之美
- 全面解析与购车指南
- 深入了解标志206最新报价,购车指南与市场分析
- 深入了解 i3 10100,一款适合日常生活的高效处理器
- 走进vivo手机商城,探索智能生活的新篇章
- 5万以下汽车报价大全,为您精选高性价比的经济型车型
- 一辆小车的精彩故事
- 全面解析与购车建议
- 深入了解昊锐1.8T油耗表现及其优化技巧
- 迈腾18T,都市出行的理想伙伴,轻松驾驭每一段旅程
- 桑塔纳新款,传承经典,焕发新生
- 联发科MT6765,智能手机的高效心脏
- 丰田Previa,一款经典MPV的前世今生
- 小学校长受贿近千万,背后的故事与启示
- 探索移动帝国论坛,连接全球移动技术爱好者的桥梁
- 小小的我预售破4000万,一场梦幻童话的奇迹之旅
- 深度解析凯迪拉克CTS(进口),豪华与性能的完美结合
- 揭秘南方人为何更易患鼻咽癌?
- 豪华与性能的完美结合——价格详解及购车指南
- 我是刑警编剧专访,坚持创作初心,不惯市场之风
- 轻松掌握图标文件的奥秘
- 黄圣依在最强大脑中的高知魅力——路透背后的故事
- 微信紧急提醒,警惕木马病毒——如何防范与应对网络攻击?
- Jeep新大切诺基,经典与现代的完美融合
- 顾客用餐时打火机不慎落入锅内引发爆炸事件解析
- 解读大捷龙报价,购车前必知的关键信息
- 大学生作业中的AI气息,新时代的学习变革
- 比亚迪思锐,探索未来汽车科技的先锋
- 警惕串联他人越级走访,数人多次煽动行为终被抓获的警示
- 经典与现代的完美融合——联想ThinkPad X201,一款改变工作方式的笔记本电脑
- 北京平谷再现鸟中老虎
- 一位七旬官员的人生转折,公诉背后的故事与深思
- 财神鱼离奇死亡,男子悲痛之余做出惊人决定,起锅烧油含泪吃下
- 掌握 Flash 课件制作,从零开始的实用教程
- 蜜雪冰城的新动作,背后的战略调整与市场应对
- 警惕网络谣言,重庆小女孩急需救助的真相揭秘
- 深入了解2012款锋范,经典小车的完美演绎
- 刘诗诗,淡然面对传闻,专注自我成长