您所在的位置:首页 - 科普 - 正文科普
链表怎么写
沅航
04-30
【科普】
851人已围观
摘要##使用Python实现链表数据结构在Python中,可以使用类来实现链表数据结构。下面是一个简单的示例,演示了如何定义一个节点类和链表类,并实现一些基本的操作。```pythonclassNode:
使用Python实现链表数据结构
在Python中,可以使用类来实现链表数据结构。下面是一个简单的示例,演示了如何定义一个节点类和链表类,并实现一些基本的操作。
```python
class Node:
def __init__(self, data):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
def append(self, data):
new_node = Node(data)
if not self.head:
self.head = new_node
else:
last_node = self.head
while last_node.next:
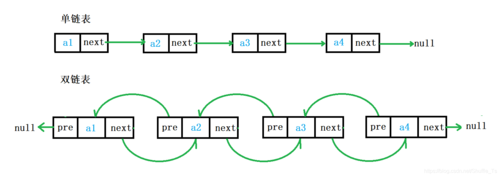
last_node = last_node.next
last_node.next = new_node
def prepend(self, data):
new_node = Node(data)
new_node.next = self.head
self.head = new_node
def delete(self, data):
current_node = self.head
if current_node and current_node.data == data:
self.head = current_node.next
current_node = None
return
prev = None
while current_node and current_node.data != data:
prev = current_node
current_node = current_node.next
if current_node is None:
return
prev.next = current_node.next
current_node = None
def display(self):
current_node = self.head
while current_node:
print(current_node.data, end=' > ')
current_node = current_node.next
print('None')
```
使用这个链表类可以进行一些操作,例如:
```python
创建链表实例
my_list = LinkedList()
向链表尾部添加元素
my_list.append(1)
my_list.append(2)
my_list.append(3)
向链表头部添加元素
my_list.prepend(4)
删除指定元素
my_list.delete(2)
显示链表内容
my_list.display()
```
以上代码演示了一个简单的链表实现,当然,链表还有很多其他操作,比如插入、查找等。这里只是提供了一个基本的框架,你可以根据需要进一步扩展链表类的功能。
希望这能帮助到你,如果有其他问题,欢迎继续提问!
参考资料
1. https://realpython.com/linkedlistspython/implementingasimplelinkedlist
2. https://www.geeksforgeeks.org/linkedlistset1introduction/
Tags: 终结者创世纪 人族无敌攻略 盗墓迷城2 江南百景图严大人交换表 真三国无双6攻略
版权声明: 免责声明:本网站部分内容由用户自行上传,若侵犯了您的权益,请联系我们处理,谢谢!联系QQ:2760375052
上一篇: api编程语言
下一篇: 学哪种编程语言更省钱呢
最近发表
- 一款值得信赖的全能座驾
- Jeep牧马人,越野传奇的全面解析
- 轻松掌握 XP 中文语言包下载与安装全攻略
- 深入探索Google操作系统,如何改变我们的数字生活
- 一款独特的美式SUV
- 轻松入门电脑知识,畅游数字世界——电脑知识学习网带你全面掌握
- 深入解读vivo Y93手机参数,性能、功能与用户体验
- 电源已接通但未充电?别慌!详解及解决方法
- 苹果SE4上市时间及价格全解析,性价比之王的回归
- 探寻AM3平台的最佳CPU选择
- 别克君威价格全解析,购车必备指南
- 全面解析与深度评测
- 理解负指数分布图像,隐藏在日常生活中的数学之美
- 全面解析与购车指南
- 深入了解标志206最新报价,购车指南与市场分析
- 深入了解 i3 10100,一款适合日常生活的高效处理器
- 走进vivo手机商城,探索智能生活的新篇章
- 5万以下汽车报价大全,为您精选高性价比的经济型车型
- 一辆小车的精彩故事
- 全面解析与购车建议
- 深入了解昊锐1.8T油耗表现及其优化技巧
- 迈腾18T,都市出行的理想伙伴,轻松驾驭每一段旅程
- 桑塔纳新款,传承经典,焕发新生
- 联发科MT6765,智能手机的高效心脏
- 丰田Previa,一款经典MPV的前世今生
- 小学校长受贿近千万,背后的故事与启示
- 探索移动帝国论坛,连接全球移动技术爱好者的桥梁
- 小小的我预售破4000万,一场梦幻童话的奇迹之旅
- 深度解析凯迪拉克CTS(进口),豪华与性能的完美结合
- 揭秘南方人为何更易患鼻咽癌?
- 豪华与性能的完美结合——价格详解及购车指南
- 我是刑警编剧专访,坚持创作初心,不惯市场之风
- 轻松掌握图标文件的奥秘
- 黄圣依在最强大脑中的高知魅力——路透背后的故事
- 微信紧急提醒,警惕木马病毒——如何防范与应对网络攻击?
- Jeep新大切诺基,经典与现代的完美融合
- 顾客用餐时打火机不慎落入锅内引发爆炸事件解析
- 解读大捷龙报价,购车前必知的关键信息
- 大学生作业中的AI气息,新时代的学习变革
- 比亚迪思锐,探索未来汽车科技的先锋
- 警惕串联他人越级走访,数人多次煽动行为终被抓获的警示
- 经典与现代的完美融合——联想ThinkPad X201,一款改变工作方式的笔记本电脑
- 北京平谷再现鸟中老虎
- 一位七旬官员的人生转折,公诉背后的故事与深思
- 财神鱼离奇死亡,男子悲痛之余做出惊人决定,起锅烧油含泪吃下
- 掌握 Flash 课件制作,从零开始的实用教程
- 蜜雪冰城的新动作,背后的战略调整与市场应对
- 警惕网络谣言,重庆小女孩急需救助的真相揭秘
- 深入了解2012款锋范,经典小车的完美演绎
- 刘诗诗,淡然面对传闻,专注自我成长