您所在的位置:首页 - 生活 - 正文生活
编程软件scratch
臻娴
04-28
【生活】
110人已围观
摘要**Title:UnderstandingtheRoleandImportanceofSwitchStatementsinProgramming**Switchstatementsareessenti
Title: Understanding the Role and Importance of Switch Statements in Programming
Switch statements are essential constructs in programming languages that allow developers to execute different code blocks based on the value of a variable or expression. Let's delve into what switch statements are, how they work, and why they are important across various programming languages.
What are Switch Statements?
Switch statements, also known as switchcase statements, provide an efficient way to perform multiple conditional branches based on the value of an expression. Instead of writing multiple ifelse statements for each possible value, a switch statement offers a more concise and readable alternative.
In most programming languages, a switch statement consists of the following components:
1.
Switch Expression
: The variable or expression whose value is evaluated.2.
Case Labels
: Values against which the switch expression is compared.3.
Code Blocks
: Executable code associated with each case label.4.
Default Case (Optional)
: Code to be executed when none of the case labels match the switch expression.How Switch Statements Work
1.
Evaluation
: The switch expression is evaluated once.2.
Comparison
: The value of the switch expression is compared with each case label.3.
Execution
: When a match is found, the corresponding code block is executed.4.
Exit
: After executing the code block, control typically exits the switch statement. However, if a break statement is not present, execution continues to the next case.Importance of Switch Statements
1.
Readability
: Switch statements enhance code readability, especially when dealing with multiple conditional branches.2.
Performance
: Switch statements can be more efficient than long chains of ifelse statements, particularly when optimizing for speed.3.
Maintainability
: They make code easier to maintain and modify, as adding or removing cases is straightforward.4.
Pattern Matching
: Switch statements are often used for pattern matching, where different actions are taken based on the input pattern.5.
Error Handling
: They are useful for error handling, allowing developers to handle different error conditions efficiently.6.
Code Organization
: Switch statements help in organizing code logically, especially when dealing with enums or constants.Best Practices for Using Switch Statements
1.
Include a Default Case
: Always include a default case to handle unexpected values or edge cases.2.
Use Enums or Constants
: Prefer using enums or constants as case labels to improve code clarity and maintainability.3.
Avoid Fallthrough
: Unless intentional, use break statements to prevent fallthrough between cases.4.
Keep it Simple
: Avoid complex logic within switch statements. If necessary, refactor the code into separate functions or methods.5.
Consider Alternatives
: In some cases, using polymorphism or other control structures like ifelseif chains might be more appropriate than switch statements.Examples Across Programming Languages
Java:
```java
int day = 3;
String dayName;
switch (day) {
case 1:
dayName = "Monday";
break;
case 2:
dayName = "Tuesday";
break;
case 3:
dayName = "Wednesday";
break;
default:
dayName = "Invalid day";
break;
}
System.out.println(dayName); // Output: Wednesday
```
Python:
```python
day = 3
day_name = {
1: "Monday",
2: "Tuesday",
3: "Wednesday"
}.get(day, "Invalid day")
print(day_name) Output: Wednesday
```
C :
```cpp
include
using namespace std;
int main() {
int day = 3;
string dayName;
switch (day) {
case 1:
dayName = "Monday";
break;
case 2:
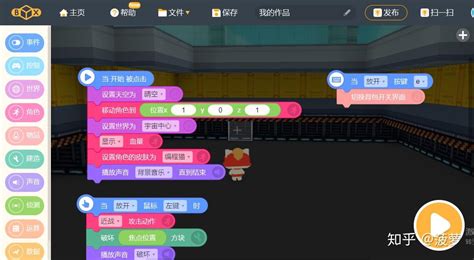
dayName = "Tuesday";
break;
case 3:
dayName = "Wednesday";
break;
default:
dayName = "Invalid day";
break;
}
cout << dayName; // Output: Wednesday
return 0;
}
```
In conclusion, switch statements are powerful tools in a programmer's arsenal, offering a structured and efficient way to handle multiple conditional branches. By understanding their syntax, functionality, and best practices, developers can leverage switch statements effectively across various programming languages to write clean, maintainable, and efficient code.
版权声明: 免责声明:本网站部分内容由用户自行上传,若侵犯了您的权益,请联系我们处理,谢谢!联系QQ:2760375052
上一篇: plsql执行按钮在哪
下一篇: 白光触摸屏报警系统错误
最近发表
- 一款值得信赖的全能座驾
- Jeep牧马人,越野传奇的全面解析
- 轻松掌握 XP 中文语言包下载与安装全攻略
- 深入探索Google操作系统,如何改变我们的数字生活
- 一款独特的美式SUV
- 轻松入门电脑知识,畅游数字世界——电脑知识学习网带你全面掌握
- 深入解读vivo Y93手机参数,性能、功能与用户体验
- 电源已接通但未充电?别慌!详解及解决方法
- 苹果SE4上市时间及价格全解析,性价比之王的回归
- 探寻AM3平台的最佳CPU选择
- 别克君威价格全解析,购车必备指南
- 全面解析与深度评测
- 理解负指数分布图像,隐藏在日常生活中的数学之美
- 全面解析与购车指南
- 深入了解标志206最新报价,购车指南与市场分析
- 深入了解 i3 10100,一款适合日常生活的高效处理器
- 走进vivo手机商城,探索智能生活的新篇章
- 5万以下汽车报价大全,为您精选高性价比的经济型车型
- 一辆小车的精彩故事
- 全面解析与购车建议
- 深入了解昊锐1.8T油耗表现及其优化技巧
- 迈腾18T,都市出行的理想伙伴,轻松驾驭每一段旅程
- 桑塔纳新款,传承经典,焕发新生
- 联发科MT6765,智能手机的高效心脏
- 丰田Previa,一款经典MPV的前世今生
- 小学校长受贿近千万,背后的故事与启示
- 探索移动帝国论坛,连接全球移动技术爱好者的桥梁
- 小小的我预售破4000万,一场梦幻童话的奇迹之旅
- 深度解析凯迪拉克CTS(进口),豪华与性能的完美结合
- 揭秘南方人为何更易患鼻咽癌?
- 豪华与性能的完美结合——价格详解及购车指南
- 我是刑警编剧专访,坚持创作初心,不惯市场之风
- 轻松掌握图标文件的奥秘
- 黄圣依在最强大脑中的高知魅力——路透背后的故事
- 微信紧急提醒,警惕木马病毒——如何防范与应对网络攻击?
- Jeep新大切诺基,经典与现代的完美融合
- 顾客用餐时打火机不慎落入锅内引发爆炸事件解析
- 解读大捷龙报价,购车前必知的关键信息
- 大学生作业中的AI气息,新时代的学习变革
- 比亚迪思锐,探索未来汽车科技的先锋
- 警惕串联他人越级走访,数人多次煽动行为终被抓获的警示
- 经典与现代的完美融合——联想ThinkPad X201,一款改变工作方式的笔记本电脑
- 北京平谷再现鸟中老虎
- 一位七旬官员的人生转折,公诉背后的故事与深思
- 财神鱼离奇死亡,男子悲痛之余做出惊人决定,起锅烧油含泪吃下
- 掌握 Flash 课件制作,从零开始的实用教程
- 蜜雪冰城的新动作,背后的战略调整与市场应对
- 警惕网络谣言,重庆小女孩急需救助的真相揭秘
- 深入了解2012款锋范,经典小车的完美演绎
- 刘诗诗,淡然面对传闻,专注自我成长