您所在的位置:首页 - 科普 - 正文科普
python多进程计算框架
贝华
2024-04-19
【科普】
901人已围观
摘要**Title:ExploringMultiprocessinginPythonforEfficientParallelComputing**MultiprocessinginPythonisapow
Title: Exploring Multiprocessing in Python for Efficient Parallel Computing
Multiprocessing in Python is a powerful tool for parallel computing, enabling the execution of multiple processes simultaneously to enhance performance and efficiency. In this guide, we'll delve into the fundamentals of multiprocessing in Python, discussing its benefits, implementation, common use cases, and best practices.
Introduction to Multiprocessing
Multiprocessing allows Python programs to execute multiple processes concurrently, taking advantage of multiple CPU cores. Unlike multithreading, which operates within a single process, multiprocessing involves separate memory spaces, making it ideal for CPUbound tasks.
Benefits of Multiprocessing
1.
Enhanced Performance
: By distributing tasks across multiple processes, multiprocessing can significantly reduce execution time, particularly for CPUintensive operations.2.
Improved Scalability
: Multiprocessing facilitates the utilization of multiple CPU cores, enabling applications to scale efficiently with hardware resources.3.
Isolation
: Each process operates independently, mitigating risks associated with shared state and resource contention, enhancing program stability.Implementation of Multiprocessing in Python
Python's `multiprocessing` module provides a straightforward interface for implementing multiprocessing functionality.
```python
import multiprocessing
def task_function(task_arg):
Task implementation
pass
if __name__ == "__main__":
Create a pool of processes
pool = multiprocessing.Pool()
Distribute tasks across processes
results = pool.map(task_function, task_args)
Close the pool
pool.close()
pool.join()
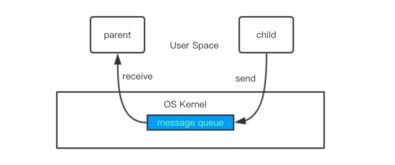
Process results
...
```
Common Use Cases
1.
Parallelizing CPUbound Tasks
: Multiprocessing is ideal for tasks that heavily utilize CPU resources, such as numerical computations, data processing, and machine learning algorithms.2.
Concurrent I/O Operations
: Although Python's Global Interpreter Lock (GIL) limits multithreading for CPUbound tasks, multiprocessing can effectively parallelize I/Obound operations, such as network requests and disk I/O.3.
Distributed Computing
: Multiprocessing enables the distribution of computational tasks across multiple nodes in a network, facilitating distributed computing for largescale applications.Best Practices for Multiprocessing
1.
Choose the Right Task Granularity
: Break tasks into appropriate chunks to maximize parallelism without incurring excessive overhead.2.
Avoid Excessive InterProcess Communication (IPC)
: Minimize communication between processes to prevent performance bottlenecks. Utilize shared memory or message passing sparingly.3.
Handle Exceptions
: Implement robust error handling to manage exceptions across multiple processes effectively.4.
Resource Management
: Be mindful of resource consumption, particularly memory usage, when spawning multiple processes.5.
Use Pooling
: Utilize process pools to manage and reuse worker processes efficiently, reducing overhead associated with process creation.Conclusion
Multiprocessing in Python empowers developers to harness the full potential of modern hardware by parallelizing computationintensive tasks. By understanding its principles, implementation, and best practices, you can leverage multiprocessing to optimize performance and scalability in your Python applications.
References:
Python Multiprocessing Documentation: [https://docs.python.org/3/library/multiprocessing.html](https://docs.python.org/3/library/multiprocessing.html)
"Effective Python: 90 Specific Ways to Write Better Python" by Brett Slatkin.
Tags: 瑞昱网卡驱动 火影忍者377 真人斗地主 梦幻西游录像
版权声明: 免责声明:本网站部分内容由用户自行上传,若侵犯了您的权益,请联系我们处理,谢谢!联系QQ:2760375052
上一篇: jquery的题库和答案
下一篇: ic芯片编程
最近发表
- 特朗普回应普京涉乌言论,强硬立场引发争议与担忧
- 民营企业如何向新而行——探索创新发展的路径与实践
- 联合国秘书长视角下的普京提议,深度解析与理解
- 广东茂名发生地震,一次轻微震动带来的启示与思考
- 刀郎演唱会外,上千歌迷的守候与共鸣
- 东北夫妻开店遭遇刁难?当地回应来了
- 特朗普惊人言论,为夺取格陵兰岛,美国不排除动用武力
- 超级食物在中国,掀起健康热潮
- 父爱无声胜有声,监控摄像头背后的温情呼唤
- 泥坑中的拥抱,一次意外的冒险之旅
- 成品油需求变天,市场趋势下的新机遇与挑战
- 警惕儿童健康隐患,10岁女孩因高烧去世背后的警示
- 提振消费,新举措助力消费复苏
- 蒙牛净利润暴跌98%的背后原因及未来展望
- 揭秘缅甸强震背后的真相,并非意外事件
- 揭秘失踪的清华毕业生罗生门背后的悲剧真相
- 冷空气终于要走了,春天的脚步近了
- 李乃文的神奇之笔,与和伟的奇妙转变
- 妹妹发现植物人哥哥离世后的崩溃大哭,生命的脆弱与情感的冲击
- 云南曲靖市会泽县发生4.4级地震,深入了解与应对之道
- 缅甸政府部门大楼倒塌事件,多名官员伤亡,揭示背后的故事
- 多方合力寻找失踪的十二岁少女,七天生死大搜寻
- S妈情绪崩溃,小S拒绝好友聚会背后的故事
- 缅甸遭遇地震,灾难之下的人间故事与影响深度解析
- 缅甸地震与瑞丽市中心高楼砖石坠落事件揭秘
- 揭秘ASP集中营,技术成长的摇篮与挑战
- 徐彬,整场高位压迫对海港形成巨大压力——战术分析与实践洞察
- ThreadX操作系统,轻量、高效与未来的嵌入式开发新选择
- 王钰栋脚踝被踩事件回应,伤势并不严重,一切都在恢复中
- 刘亦菲,粉色花瓣裙美神降临
- 三星W2018与G9298,高端翻盖手机的对比分析
- 多哈世乒赛器材,赛场内外的热议焦点
- K2两厢车,小巧灵活的城市出行神器,适合你的生活吗?
- 国家市监局将审查李嘉诚港口交易,聚焦市场关注焦点
- 提升知识水平的趣味之旅
- 清明五一档电影市场繁荣,多部影片争相上映,你期待哪一部?
- 美联储再次面临痛苦抉择,权衡通胀与经济恢复
- 家庭千万别买投影仪——真相大揭秘!
- 文物当上网红后,年轻人的创意与传承之道
- 手机解除Root的最简单方法,安全、快速、易操作
- 缅甸地震与汶川地震,能量的震撼与对比
- 2011款奥迪A8,豪华与科技的完美结合
- 广州惊艳亮相,可折叠电动垂直起降飞行器革新城市交通方式
- 比亚迪F3最低报价解析,性价比之选的购车指南
- 商业健康保险药品征求意见,行业内外视角与实用建议
- 官方动态解读,最低工资标准的合理调整
- 东风标致5008最新报价出炉,性价比杀手来了!
- 大陆配偶在台湾遭遇限期离台风波,各界发声背后的故事与影响
- 奔驰C级2022新款,豪华与科技的完美融合
- 大摩小摩去年四季度对A股的投资热潮