您所在的位置:首页 - 百科 - 正文百科
编程fortran
至东
04-18
【百科】
277人已围观
摘要IntroductiontoForLoopinProgrammingIntroductiontoForLoopinProgrammingAforloopisafundamentalconceptinp
Introduction to For Loop in Programming
A for loop is a fundamental concept in programming that allows you to repeat a block of code a certain number of times. It is commonly used when you know in advance how many times you want to execute a particular set of instructions.
The basic syntax of a for loop in most programming languages is as follows:
for (initialization; condition; increment/decrement) { // Code to be executed }
- Initialization: This is where you initialize the loop control variable. It is typically used to set the starting value of the variable.
- Condition: The loop will continue to execute as long as this condition is true. Once the condition becomes false, the loop will terminate.
- Increment/Decrement: This is used to update the loop control variable after each iteration. It can be an increment (i ) or decrement (i--).
Here is an example of a simple for loop in Python that prints numbers from 1 to 5:
for i in range(1, 6):
print(i)
In this example:
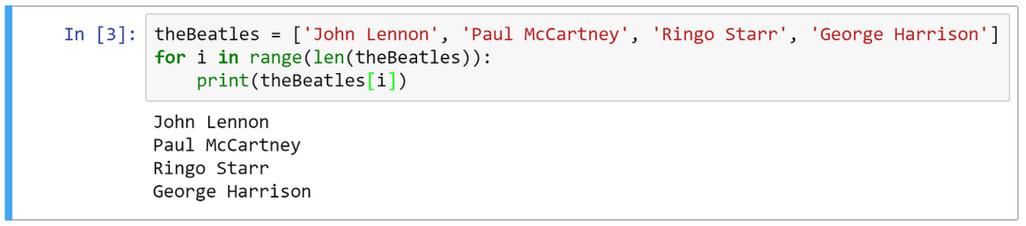
range(1, 6)
generates a sequence of numbers from 1 to 5.- The loop iterates over each number in the sequence and prints it.
For loops offer several advantages in programming:
- Readability: For loops make the code more readable and easier to understand, especially when you need to repeat a block of code a specific number of times.
- Efficiency: They help in writing concise and efficient code by reducing redundancy.
- Control: For loops provide better control over the flow of the program, allowing you to iterate over a sequence of elements with ease.
When using for loops, it is important to follow some best practices to ensure efficient and maintainable code:
- Initialize Variables Properly: Make sure to initialize loop control variables before using them in a for loop.
- Avoid Infinite Loops: Always ensure that the loop condition will eventually become false to prevent infinite loops.
- Keep the Code Inside the Loop Concise: Try to keep the code inside the loop as concise as possible to improve readability.
- Use Meaningful Variable Names: Choose meaningful names for loop control variables to enhance code clarity.
For loops are essential constructs in programming that allow you to iterate over a sequence of elements efficiently. By understanding the basic syntax, benefits, and best practices of using for loops, you can write more structured and readable code in your programs.