您所在的位置:首页 - 百科 - 正文百科
TipsforChessboardProgramminginC:
临闯
2024-04-15
【百科】
105人已围观
摘要ChessboardProgramminginCChessboardProgramminginCCreatingachessboardinCinvolvesrepresentingtheboardas
Chessboard Programming in C
Creating a chessboard in C involves representing the board as a 2D array and implementing the logic for moving the pieces. Here is a basic example of how you can approach this:
```c #includeThis code snippet initializes an 8x8 chessboard with pieces in their starting positions and defines a function to print the board. You can expand on this by adding functions to move pieces, check for valid moves, and implement the rules of chess.
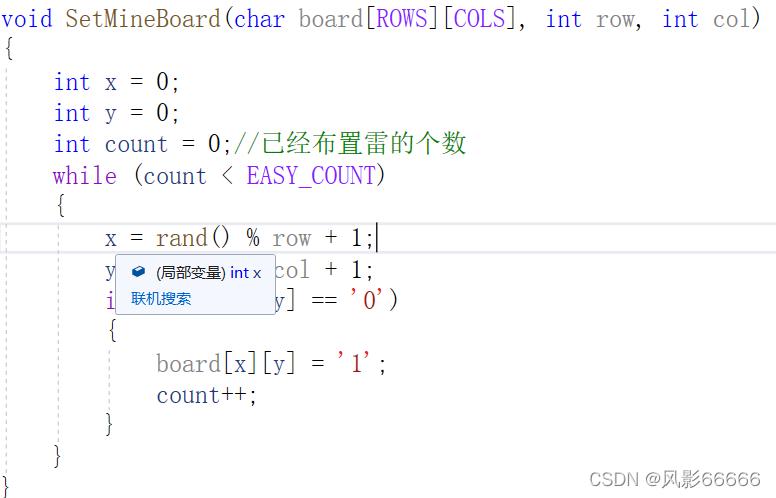
- Use a 2D Array: Represent the chessboard as a 2D array for easy manipulation of pieces.
- Implement Piece Movement: Create functions to handle moving pieces based on the rules of chess.
- Check Valid Moves: Ensure that moves are valid based on the type of piece and the current game state.
- Handle Special Moves: Implement special moves like castling, en passant, and pawn promotion.
- Consider Object-Oriented Approach: For more complex chess programs, consider using a more object-oriented approach to represent pieces and the board.
By following these tips and building on the basic example provided, you can create a functional chess program in C. Remember to test your code thoroughly and consider edge cases to ensure the correctness of your implementation.